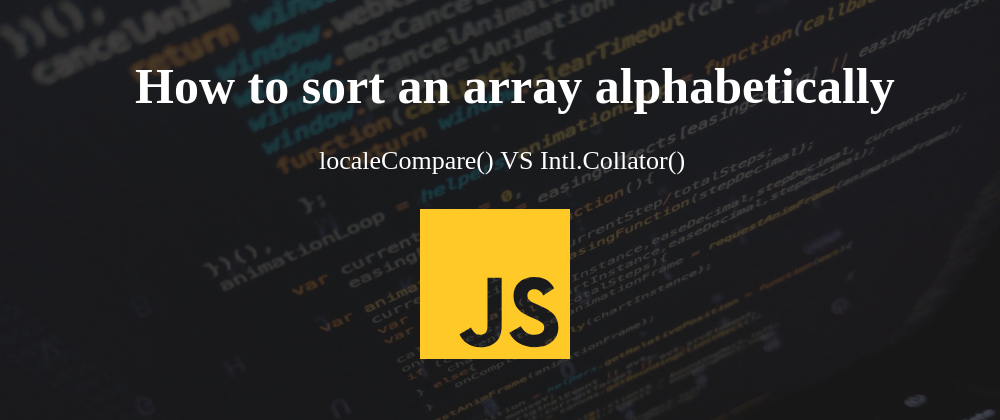
How to sort an array alphabetically
String.localeCompare()
If you're working with a relatively small array, you could use localeCompare().
const arr = [
{
name: "Orange"
},
{
name: "Banana"
},
{
name: "Carrot"
},
{
name: "Apple"
}
];
// [{"name":"Apple"},{"name":"Banana"},{"name":"Carrot"},{"name":"Orange"}]
console.log(arr.sort((a, b) => a.name.localeCompare(b.name)));
Intl.Collator()
If you're working with a large array, I would recommend using Intl.Collator() for performance reasons.
const arr = [
{
name: "Orange"
},
{
name: "Banana"
},
{
name: "Carrot"
},
{
name: "Apple"
}
];
const collator = new Intl.Collator();
// [{"name":"Apple"},{"name":"Banana"},{"name":"Carrot"},{"name":"Orange"}]
console.log(arr.sort((a, b) => collator.compare(a.name, b.name)));
Benchmarks
1,000 strings
Here is a benchmark where we're sorting an array of 1,000 strings. As you can see, Intl.Collator() is 25% faster than localeCompare().
25 strings
Here is a benchmark where we're sorting an array of only 25 strings. In this case, localeCompare() is 13% faster than Intl.Collator().