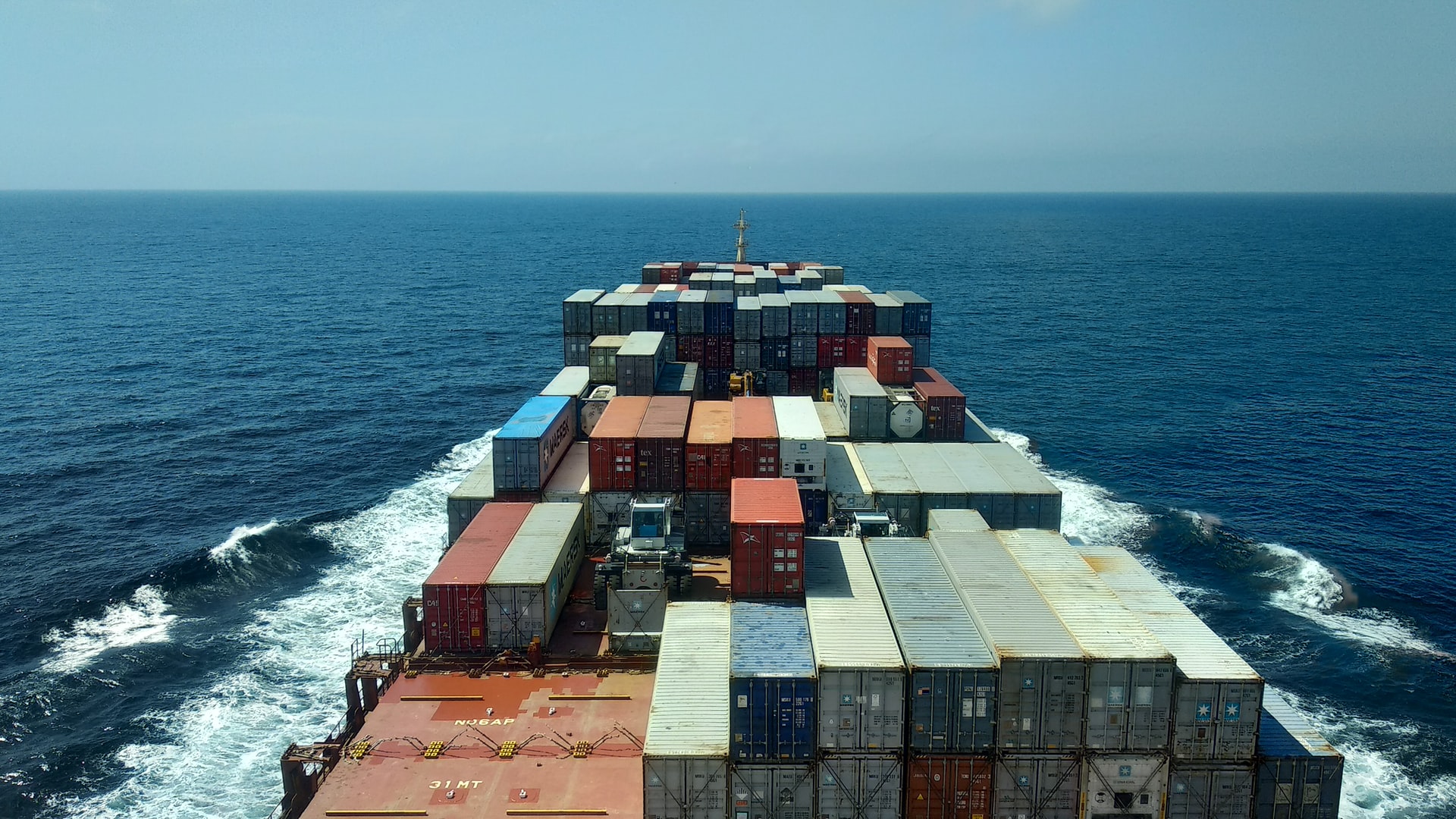
Introduction to Docker
What is Docker?
Docker allows developers to run their applications in a predefined environment. Have you ever heard the phrase
It works on my machine
?
Well, no more! You can write a Dockerfile that packages up all the dependencies and configurations someone might need to run your application.
Docker is open-source software so anyone can contribute to its development.
Terms
Here are a few terms along with my definition. You can find a more detailed glossary on Docker docs.
Dockerfile
A text file that contains all the commands/instructions to build an image. You can think of this as a template.
image
The result of building a Dockerfile (docker build -t my_image_name .
). You can think of this as your compiled template.
container
An instance of an image (docker run --name my_instance my_image_name
)
ENTRYPOINT
The command that's run within the container once the container starts.
volume
Directory on your host machine that can be mapped to a location within your container (docker run --name my_instance -v /home/adam/my-project:/var/www/html my_image_name
)
Installing Docker
Instead of walking you through the installation steps for each operating system, I'll refer you to the official Docker documentation:
Getting started
Now that you have Docker installed on your host machine you can start creating containers!
Let's run an instance of hello-world:
docker run hello-world
Creating an image
The purpose of this image will be to run a simple Apache server.
To create an image you'll need to write a Dockerfile, to do that make a new file, and name it
Dockerfile
(no file extension).
FROM ubuntu:latest
RUN apt-get update && DEBIAN_FRONTEND=noninteractive apt-get -y install apache2
EXPOSE 80
CMD /usr/sbin/apache2ctl -D FOREGROUND
Now run the following command to build your image:
docker build -t simple-apache-server .
You should see the following output:
Running a container
Now that you've built an image, let's run an instance of it.
docker run -d --name my-apache-container -p 3338:80 simple-apache-server
Navigate to localhost:3338 in your browser and you should see the default apache page!
An explanation of the different arguments used in the command above:
-d
will run the container in the background and print its container ID.-p
will publish the container's port to the host.HOST_PORT
:CONTAINER_PORT
--name
lets you assign a name to the container.
Helpful commands
List the images you've built
docker images
List running containers
docker ps
List all containers (running and stopped)
docker ps -a
Remove a container
docker rm CONTAINER_NAME
Stop a container
docker stop CONTAINER_NAME
Start a container
docker start CONTAINER_NAME
"bash into" a running container
docker exec -it CONTAINER_NAME bash