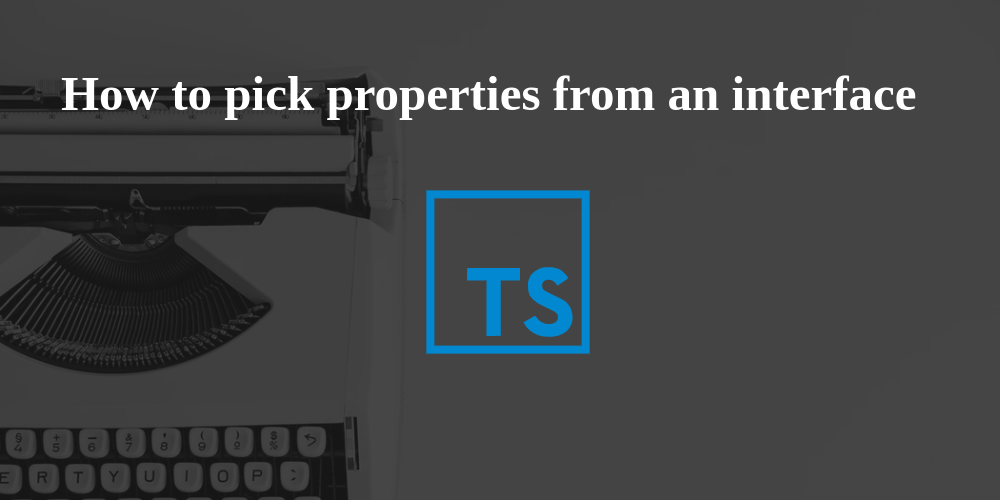
How to pick properties from an interface
You can use the built-in type Pick. Pick<MyInterface, "prop1" | "prop2">
is a built-in type which allows you to pick specific properties from an interface.
A great use-case would be verifying user credentials. You might have a User
interface such as the following:
interface User {
id: number;
firstName: string;
lastName: string;
email: string;
password: string;
}
Instead of creating a new interface with the
email
and password
properties from the
User
interface, lets Pick
them!
// without Pick
async function verifyLoginCredentials(credentials: { email: string, password: string }) {
// ... ...
}
// with Pick
async function verifyLoginCredentials(credentials: Pick<User, "email" | "password">) {
const user = await getUserByEmail(credentials.email);
if (!passwordMatches(credentials.password, user.password)) {
throw new BadRequestError('incorrect password');
}
return user;
}
verifyLoginCredentials({ email: "[email protected]", password: "123" });